welcome to tips4you
Ques: Develop a simple application, which will create a server and a client using the sockets interface.
The server waits for TCP connections on TCP port 15710. When a client establishes a connection to that
port, the server waits for the client to initiate communication.
The information exchange between client and server proceeds as follows:
a) The client program sends a request message to the server, identifying itself (Its IP address, and port)
b) The server then sends a line of text to the client containing a random number of characters.
c) The client sends back a number equal to the number of characters in the line of text and closes its end of the connection. So if the server sent 132 bytes of text, the client would send ``132''.
The server sends back a response indicating whether the client's input was correct.
Ques: Develop a simple application, which will create a server and a client using the sockets interface.
The server waits for TCP connections on TCP port 15710. When a client establishes a connection to that
port, the server waits for the client to initiate communication.
The information exchange between client and server proceeds as follows:
a) The client program sends a request message to the server, identifying itself (Its IP address, and port)
b) The server then sends a line of text to the client containing a random number of characters.
c) The client sends back a number equal to the number of characters in the line of text and closes its end of the connection. So if the server sent 132 bytes of text, the client would send ``132''.
The server sends back a response indicating whether the client's input was correct.
Source Code:
Server:
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.ServerSocket;
import java.net.Socket;
public class Server {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(15710);
System.out.println("Waiting for Client");
Socket client = serverSocket.accept();
handleClient(client);
}
private static void handleClient(Socket client) throws IOException {
System.out.println("Client Ip : "+client.getInetAddress().getHostAddress());
System.out.println("Client Port : "+client.getPort());
BufferedReader inputStream = new BufferedReader(new InputStreamReader(client.getInputStream()));
BufferedOutputStream outputStream = new BufferedOutputStream(client.getOutputStream());
String text = inputStream.readLine();
System.out.println("Received String : "+text);
outputStream.write((text.length()+"\n").getBytes());
outputStream.flush();
System.out.println("Sent Message : "+text.length());
inputStream.close();
outputStream.close();
client.close();
}
}
Client:
import java.io.*;
import java.net.Socket;
public class Client {
public static void main(String[] args) throws IOException {
Socket socket = new Socket("127.0.0.1",15710);
System.out.println("Connected To Server");
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
BufferedOutputStream outputStream = new BufferedOutputStream(socket.getOutputStream());
String x = "fuwefiewfi fwefiwofwofowiwihwrri4 r4irwriowroirwjwoow";
outputStream.write((x+"\n").getBytes());
outputStream.flush();
System.out.println("Sent String : "+x);
int y = Integer.parseInt(in.readLine());
System.out.println("Received Message : "+y);
socket.close();
}
}
Output:
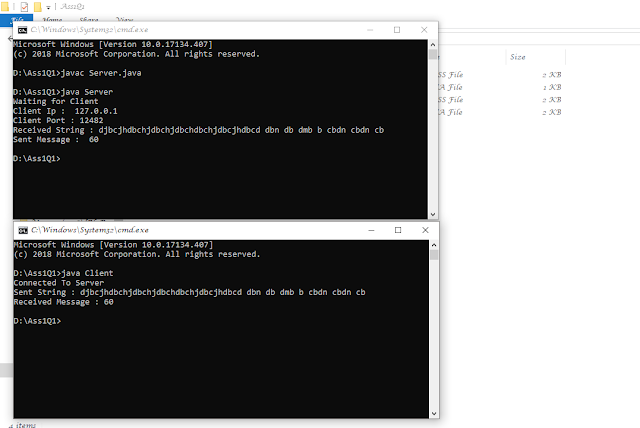
Develop a simple application, which will create a server and a client using the sockets interface. client server socket programming in java java socket client example socket programming in java source code udp client server program in java tutorial socket programming in java pdf chat application in java using socket programming how to get client port number in server socket programming in java client server socket programming in c
Comments