Ques: Use TCP sockets to create a client-server application, which behaves as follows:
Two different clients send an integer value to the server. The server receives these values from both the client's and sends back the sum of the two values supplied by the two clients. After the first client
connects, the server will wait for the second client to connect. The server must support multiple connections at a time. The server will read integer values from both the clients and send back the sum of
these two values to both the clients.
Two different clients send an integer value to the server. The server receives these values from both the client's and sends back the sum of the two values supplied by the two clients. After the first client
connects, the server will wait for the second client to connect. The server must support multiple connections at a time. The server will read integer values from both the clients and send back the sum of
these two values to both the clients.
Source Code:
Server:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.ServerSocket;
import java.net.Socket;
public class Server {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(1845);
System.out.println("Waiting For Clients");
Socket clientOne = serverSocket.accept();
System.out.println("First Client Connected From Port : "+clientOne.getPort());
Socket clientTwo = serverSocket.accept();
System.out.println("First Client Connected From Port : "+clientTwo.getPort());
BufferedReader brone= new BufferedReader(new InputStreamReader(clientOne.getInputStream()));
BufferedReader brtwo = new BufferedReader(new InputStreamReader(clientTwo.getInputStream()));
int x = Integer.parseInt(brone.readLine());
System.out.println("First Client Sent : "+x);
int y = Integer.parseInt(brtwo.readLine());
System.out.println("Second Client Sent : "+y);
OutputStream osone = clientOne.getOutputStream();
OutputStream ostwo = clientTwo.getOutputStream();
osone.write(((x+y)+"\n").getBytes());
osone.flush();
ostwo.write(((x+y)+"\n").getBytes());
ostwo.flush();
clientOne.close();
clientTwo.close();
serverSocket.close();
}
}
Client:
import java.io.*;
import java.net.Socket;
import java.util.Random;
public class Client {
public static void main(String[] args) throws IOException {
Socket socket = new Socket("127.0.0.1",1845);
System.out.println("Connected To Server");
int random = new Random().nextInt();
OutputStream os = socket.getOutputStream();
os.write((random+"\n").getBytes());
os.flush();
System.out.println("Sent : "+random);
BufferedReader br = new BufferedReader(new InputStreamReader(socket.getInputStream()));
long sum = Long.parseLong(br.readLine());
System.out.println("Received Sum : "+sum);
socket.close();
}
}
Output:
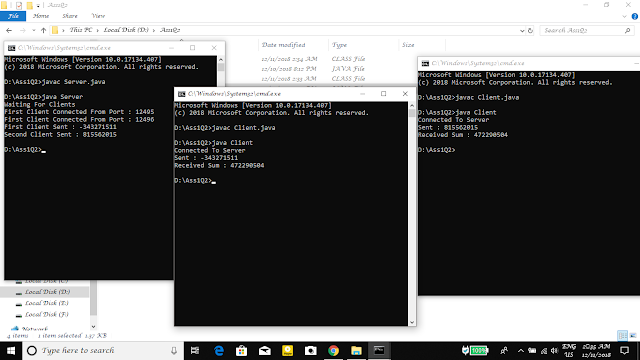
Comments