Ques: Use TCP sockets to build a simple client-server system, where you use the client to chat with a dummy server. The protocol between the client and server is as 📍 :
a) The server is first started on a known port.
b) The client program is started (server IP and port are provided on the command line).
c) The client connects to the server, and then asks the user for input. The user types his message on the terminal (as "Bye", "How are you","Hi"). The user's input is sent to the server via the connected socket.
d) The server reads the user's input from the client socket. If the user has typed "Bye" (without " "), the server must reply with "Goodbye". For other messages, server must reply with OK.
e) The client then reads the reply from the server, and checks that it is accurate (either "OK" or "Goodbye").
f) If user type "Bye", and the server reply with a "Goodbye" correctly, the client quits. Otherwise, client asks user forthe next message to send to the server.
If the server sends a wrong reply, say, "NO" instead of "OK", the client exits with an error message.
a) The server is first started on a known port.
b) The client program is started (server IP and port are provided on the command line).
c) The client connects to the server, and then asks the user for input. The user types his message on the terminal (as "Bye", "How are you","Hi"). The user's input is sent to the server via the connected socket.
d) The server reads the user's input from the client socket. If the user has typed "Bye" (without " "), the server must reply with "Goodbye". For other messages, server must reply with OK.
e) The client then reads the reply from the server, and checks that it is accurate (either "OK" or "Goodbye").
f) If user type "Bye", and the server reply with a "Goodbye" correctly, the client quits. Otherwise, client asks user forthe next message to send to the server.
If the server sends a wrong reply, say, "NO" instead of "OK", the client exits with an error message.
Source Code:
Server:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.ServerSocket;
import java.net.Socket;
public class Server {
public static void main(String[] args) throws IOException {
ServerSocket socket = new ServerSocket(7458);
System.out.println("Waiting For Client");
Socket client = socket.accept();
handleSocket(client);
}
private static void handleSocket(Socket client) throws IOException {
System.out.println("Client Connected From Port : "+client.getPort());
BufferedReader in = new BufferedReader(new InputStreamReader(client.getInputStream()));
PrintWriter out = new PrintWriter(client.getOutputStream());
while (true){
String msg = in.readLine().trim();
System.out.println("Client : "+msg);
if (msg.equalsIgnoreCase("bye")){
out.println("Goodbye");
out.flush();
client.close();
System.out.println("Server : Goodbye");
break;
} else {
out.println("OK");
out.flush();
}
}
}
}
Client:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.Socket;
public class Client {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Enter Ip Address: ");
String ip = br.readLine();
System.out.println("Enter Port :");
int port = Integer.parseInt(br.readLine());
Socket socket = new Socket(ip,port);
System.out.println("Connected To Server");
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream());
while (true){
System.out.println("Message: ");
String x = br.readLine();
out.println(x);
out.flush();
String y = in.readLine();
if (y.trim().equalsIgnoreCase("goodbye")){
System.out.println("Closing Client");
socket.close();
break;
} else {
System.out.println("Server : "+y);
}
}
}
}
Output:
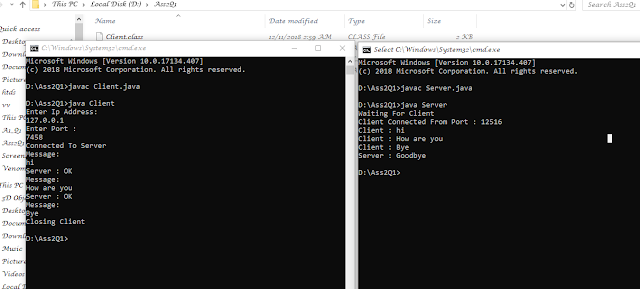
Comments